Become 100% job ready with our Core Java course!!
Let us guide you!
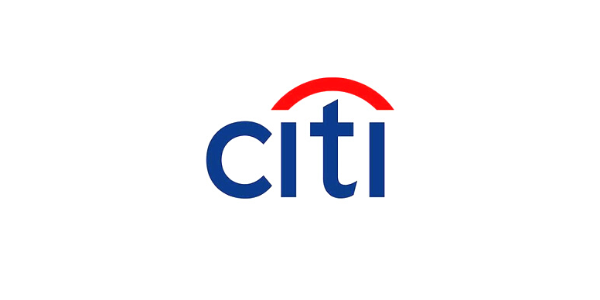
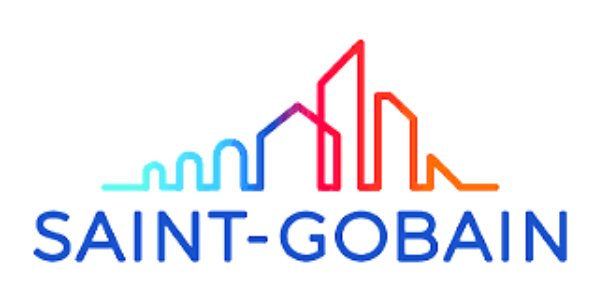
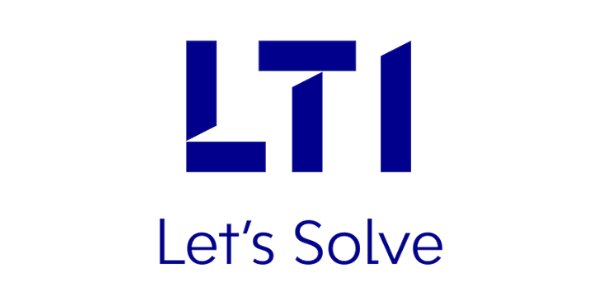
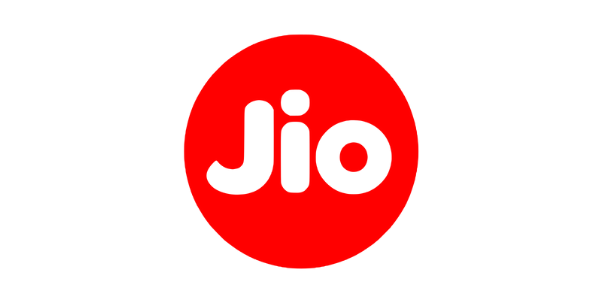
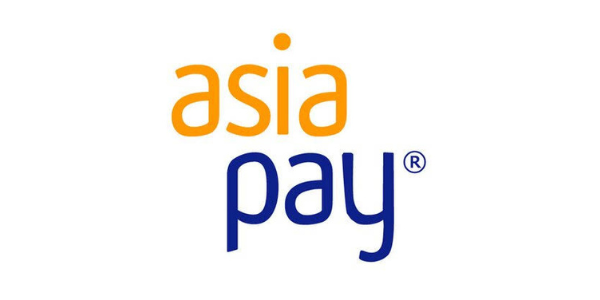
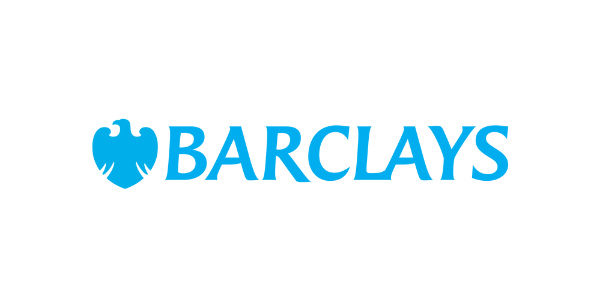
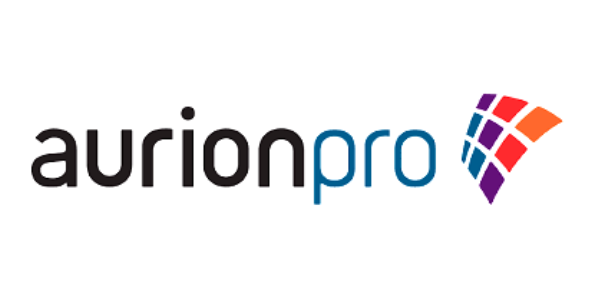
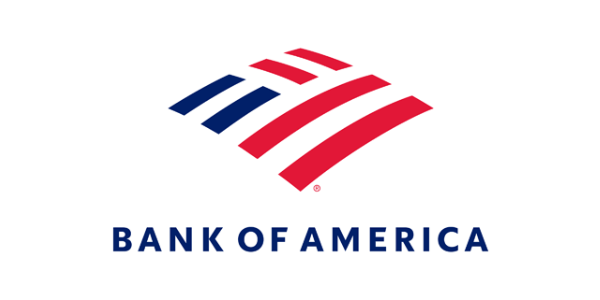
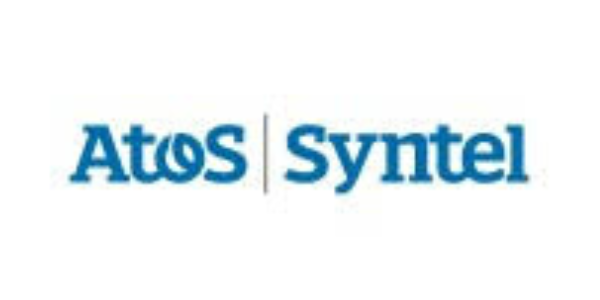
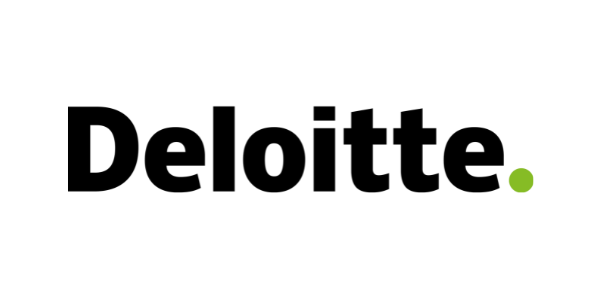
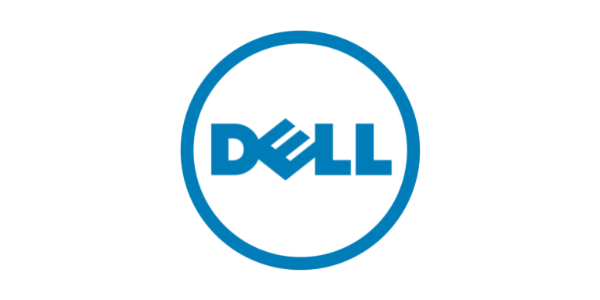
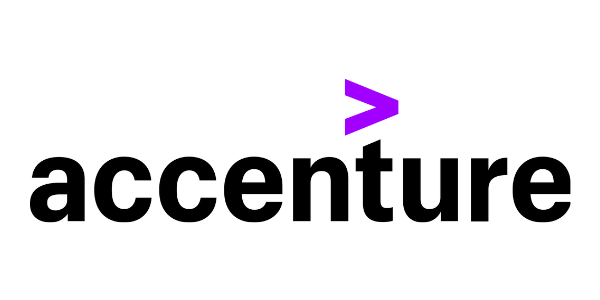
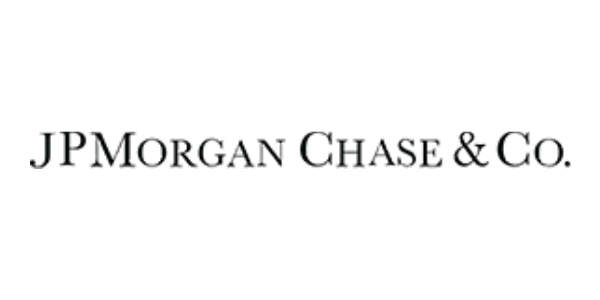
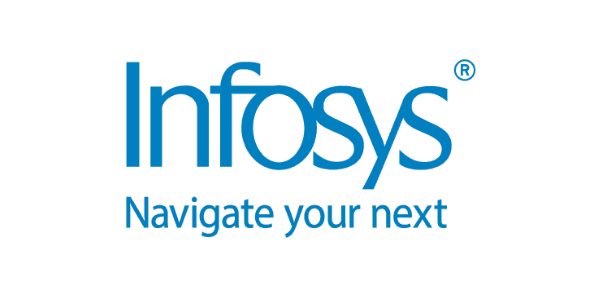
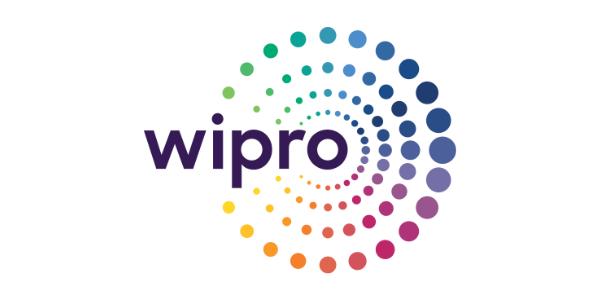
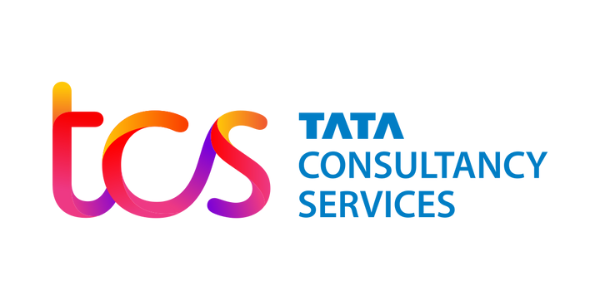
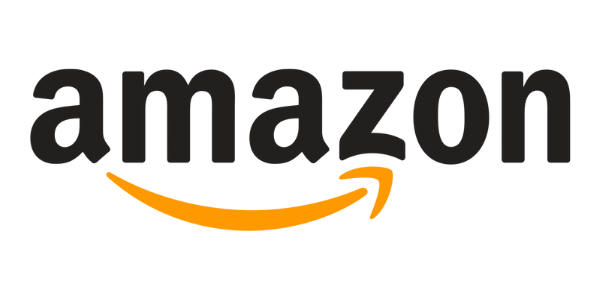
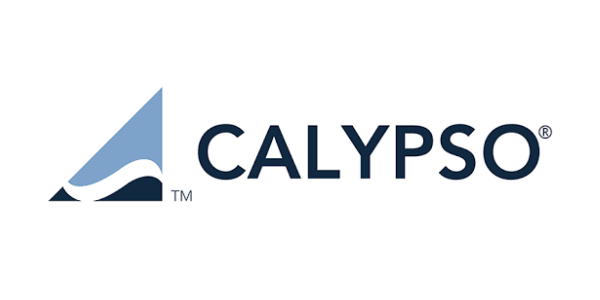
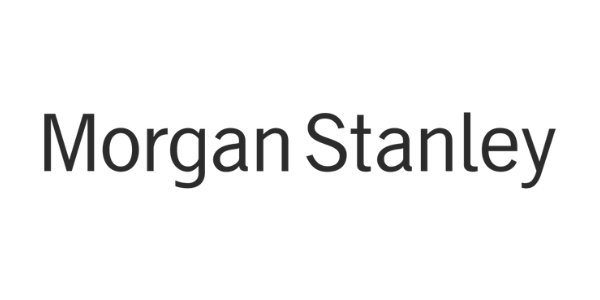
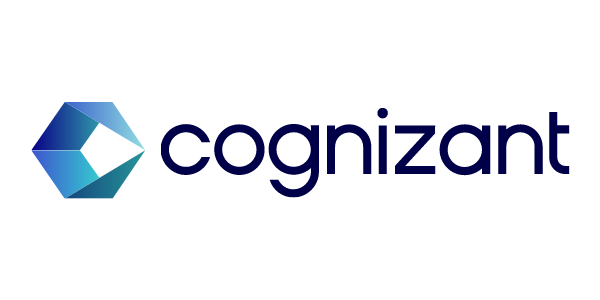
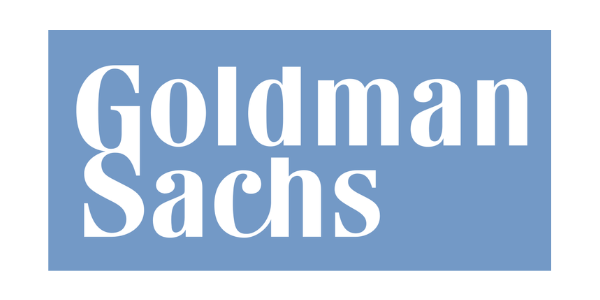
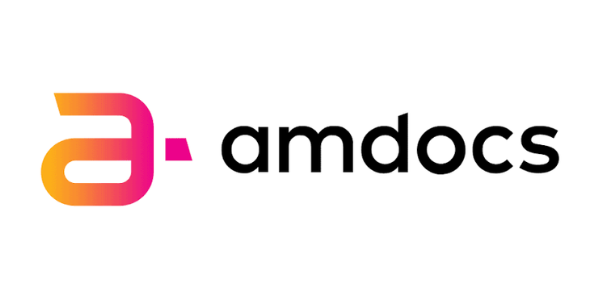
Core Java Curriculum
2.5 Months
Practical training
Major Projects!
OOP and Java Language Architecture
- How to model Real World Objects in Software.
- Understanding similarities between real world and software world in OOP.
- Understanding how objects interact (Notion of Interfaces).
- What is a class and how to write a class in Java.
- Why classes are called abstract data types.
- Understanding inheritance, overriding and overloading.
- Understanding difference between reference variables and objects.
- Understanding what is a reference to an object.
- How to instantiate objects in Java.
- Understanding Constructors.
- No destructors in Java. It’s Garbage Collector.
- Runtime Polymorphism.
- Understanding compiler works on data types.
- Rules and conventions in Java.
- Understanding instance variables & instance methods .Why are they called so.
- Understanding class (static) variables & class (static) methods why are they called so.
- Why main method is static.
- Installing JDK & Edit Plus (Editor).
- Home Directory of Java.
- Doing settings for JAVA_HOME, path & classpath environment variables.
- Understanding path.
- Writing a program and proving all the concepts we learnt in Lecture 1.
- (PRACTICAL)
- History of Java.
- Official definition of Java Language.
- Architecture of Java .(Source Code , Byte Code, JVM).
- Why the name JVM.
- Does JVM really exists.
- JIT Compiler what is it. Why used?
- Understanding main method is not a syntactical compulsion it’s a logical requirement.
- Do we need to have main method in public class let’s answer that?
- Primitive Data Types Supported by Java and corresponding Wrapper Classes.
Java Library, Access Modifiers, Packaging
- What makes up Java Library.
- Where is it?
- How is it organized (Packages).
- Why Object class is the most important class in Java.
- Java supports Multilevel Inheritance in classes why so?
- What is classpath environment variable.
- How to use the Library.
- The 4 access modifiers in Java. What is the difference in access?
- Protected Access Modifier hides something from you lets expose it.
- Practical:- Exposing Protected Access Modifier.
- Practical:- How to Package our class files.
Applets
- What is an Applet?
- How to write an Applet.
- Life cycle methods of Applet.
- Writing Applets with GUI Components on it.
- Understanding add() method to add GUI Components.
- Applet class hierarchy.
- Event Handling using Chaining Model.
- Practical:- Writing Applets and Handling
Event using Chaining model. - Practical:- Writing more Applets with more GUI Components.
- Practical:- Understanding how chaining works in Chaining Model.
- Practical:- How to be a Container & Component.
- Understanding Why initialization in init() method of Applet & not in constructor.
- Understanding AppletContext.
- Practical:- Writing more Applets.
Event Delegation Model, Abstract Classes, Interfaces
- How to handle events using Event Delegation Model.
- Understanding Abstract Classes & Interfaces.
- Understanding Listeners (ActionListener, FocusListener, MouseMotionListener etc.).
- Understanding Importance of Abstract Classes Logically & Syntactically.
- How to write Abstract Classes & abstract methods.
- What are Interfaces?
- How to write Interfaces in Java.
- What all things can you put in an Interface.
- Multiple Inheritance in Interfaces.
- Marker Interfaces.
- Functional Interfaces (NEW IN JDK 8).
- public default methods in interfaces (NEW IN JDK 8).
- Understanding final modifier.
- Understanding How class can implement an interface.
Inner Classes, Outer Classes, Anonymous Inner Classes
- Practical:- Event Handling using Event Delegation Model.
- Practical:- Understanding & using Inner Classes.
- Practical:- Understanding and using Outer classes.
- Practical:- Understanding & using Anonymous Inner Classes.
- Practical:- Understanding and using Lambda Expressions (NEW IN JDK 8).
- Practical:- Registering ActionListener, FocusListener, MouseMotionListener.
- Understanding Adapter Classes (FocusAdapter, MouseAdapter etc.).
- Typical things about Adapter classes.
GUI Applications, Graphics
- Practical:- Writing GUI Applications. The Frame class.
- Practical:- Using FlowLayout, GridLayout,BorderLayout Managers.
- Practical:- Drawings on an Applet and Frame. The paint() method.
- Practical:- More programs including Color and Font class and repaint() method.
Arrays - Single Dimensional and Multidimensional
- Declaring & Initializing Arrays.
- Understanding Arrays are objects in Java.
- Polymorphism in Arrays.
- ArrayStoreException when does it happen.
- ArrayIndexOutOfBoundsException when does it happen.
- How objects should be compared.
- Typecasting in objects.
- What as per compiler is valid typecasting and what is not.
- What typecasting turns out to be invalid at runtime.
- How to validate typecasting using instanceof operator.
- Typecasting in primitives.
- How are multidimensional arrays implemented in Java.
- Practical:- Using arrays in applications.
Threads
- What is Multi Tasking?
- What is Multi Processing & What is Multi Threading.
- Heap Memory and Stack Memory.
- How to instantiate and start threads.
- Thread class and Runnable Interface.
- Using one Object as Thread & Runnable.
- Using separate Thread object & separate Runnable object.
- The main thread of JVM.
- Methods in Thread class.
- Practical:- creating Threads in an application.
- Practical:- Using Inner classes for Runnable.
- Practical:- Using Outer classes for Runnable.
- Practical:- Using Anonymous Inner classes for Runnable.
- Practical:- Using Lambda Expressions.
Synchronization, Inter Thread Communication, Deadlock
- Understanding Thread Syncronization.
- Practical:- Implementing Thread Synchronization.
- Understanding Inter Thread Communication (wait() & notify() methods).
- Understanding Thread States.
- Practical:- Implementing Inter Thread Communication (Producer Consumer
Problem). - Understanding deadlock.
- Difference between wait() & sleep() methods.
- Practical:- Creating deadlock and resolving it.
Exception Handling
- try, catch, throw, throws, finally in brief.
- The flow of your application when exception occurs and when it does not.
- Exception classes hierarchy.
- Checked And Unchecked Exceptions.
- The throws clause, how is it important.
- Writing your own Exceptions.
- What you can and what you can’t do with throws clause.
- How is finally different from catch.
- When Logically to use try finally & when logically to use try catch finally.
Collections Framework & Generics
- What is Collections Framework.
- When do we use it?
- Framework works on equals() & hashCode() methods. Understanding these
methods. - Framework works on Comparable & Comparator Interfaces. Understanding these
Interfaces. - Organization of Collections Framework.
- Different Data Structures and their specialties.
- Difference between Vector & ArrayList.
- Difference between Hashtable & HashSet.
- Which Data Structure works on what.
- Auto Boxing & Auto Unboxing.
- The new forEach loop.
- Generics —We needed it.
- Java’s Compromise in Generics.
- Understanding Polymorphism not supported in Parameterized types.
- Understanding why not supported.
- Wild Card (? extends).
- Wild Card (? super).
- Writing your own Generic classes & Interfaces.
- Demo:- Lets see all the things that we learnt.
- Demo:- More Demos on Collections Framework & Generics.
- Concept & practical:- Overloaded methods with super and subclass parameters.
- Concept & practical:- Variable Arguments.
- Concept & practical:- static and non static blocks.
- Concept & practical:- Enums.
IO & NIO
- Stream an abstraction in IO.
- Hierarchy of Stream Classes (InputStream & OutputStream Hierarchy).
- Basic objects and wrappers.
- Practical:- Using System.in, Using it to read Lines from Console without wrapping.
- Practical:- Wrapping System.in and using it to read Lines from console.
- Practical:- Wrapping FileInputStream and using it to read Lines from File.
- Practical:- Using File class to access properties of File and Directory.
- Practical:- Making a File Browser.
- Reader & Writer hierarchies.
- Practical:- Using Reader & Writer hierarchies for character Reading writing.
- Practical:- Using Console class for reading writing. Also Reading username &
password. - Practical:- Using Path interface(NIO).
- Practical:- Using Files class(NIO) for creating, copying, moving and deleting file.
- Practical:- Using Files class & Path interface for entire walk through file system.
Swings and Java Beans
- AWT followed by Swings.
- Swing features:- More Good Looking.
- Swing Features:- More Features.
- Swing Features:- Different Look & Feel.
- Swing Features:- MVC Design used in Swings.
- Practical:- Writing Applications and Applets using swing components.
- Understanding Java Bean Specification for Reusable Components.
- Why follow Java Bean Specification?
- Setters and Getters.
- Practical:- Developing our own Component (Round Button).
- Practical:- Using our Round Button in our own Application.
- Practical:- Registering multiple ActionListener’s with our component.
Our students have a few things to say...
Get placed in your dream company!
Become 100% Job ready to work with our alumni in the top MNCs!
Let’s pursue your dream together!
Let us guide you!
Testimonials
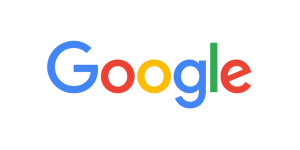
4.8 / 5
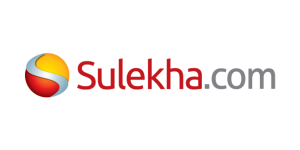
4.5 / 5
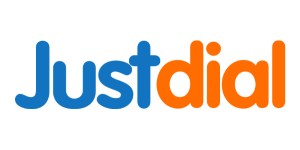
4.7 / 5
Trusted by Thousands of Students
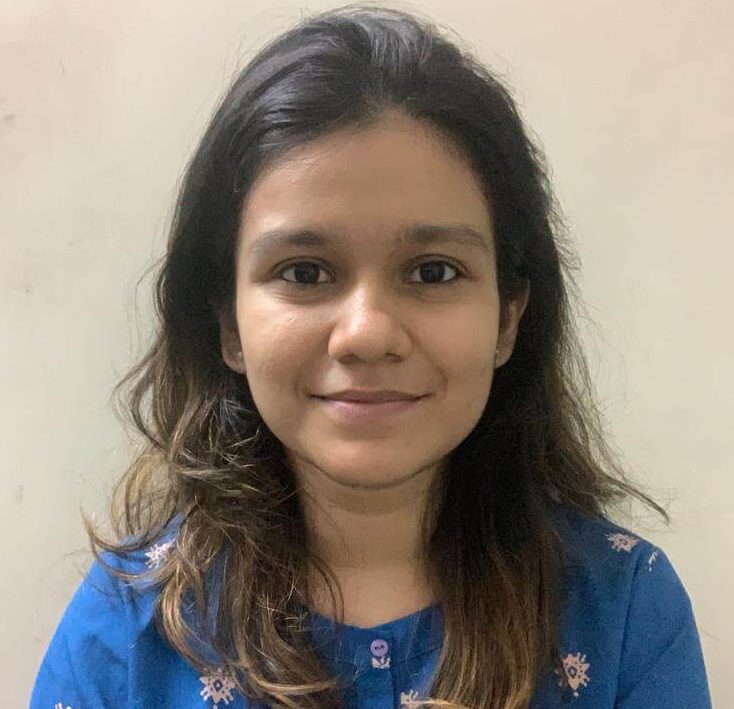
Akshaya Mulay
Accenture
Got to know about mikado from a friend and it was one of the best decisions i ever made during the starting phase of my career.
Sanjay sir’s way of teaching is just top notch and also he has a very interactive way of teaching which results into detailed learning.
Superb learing experience and great atmosphere ! 🙂
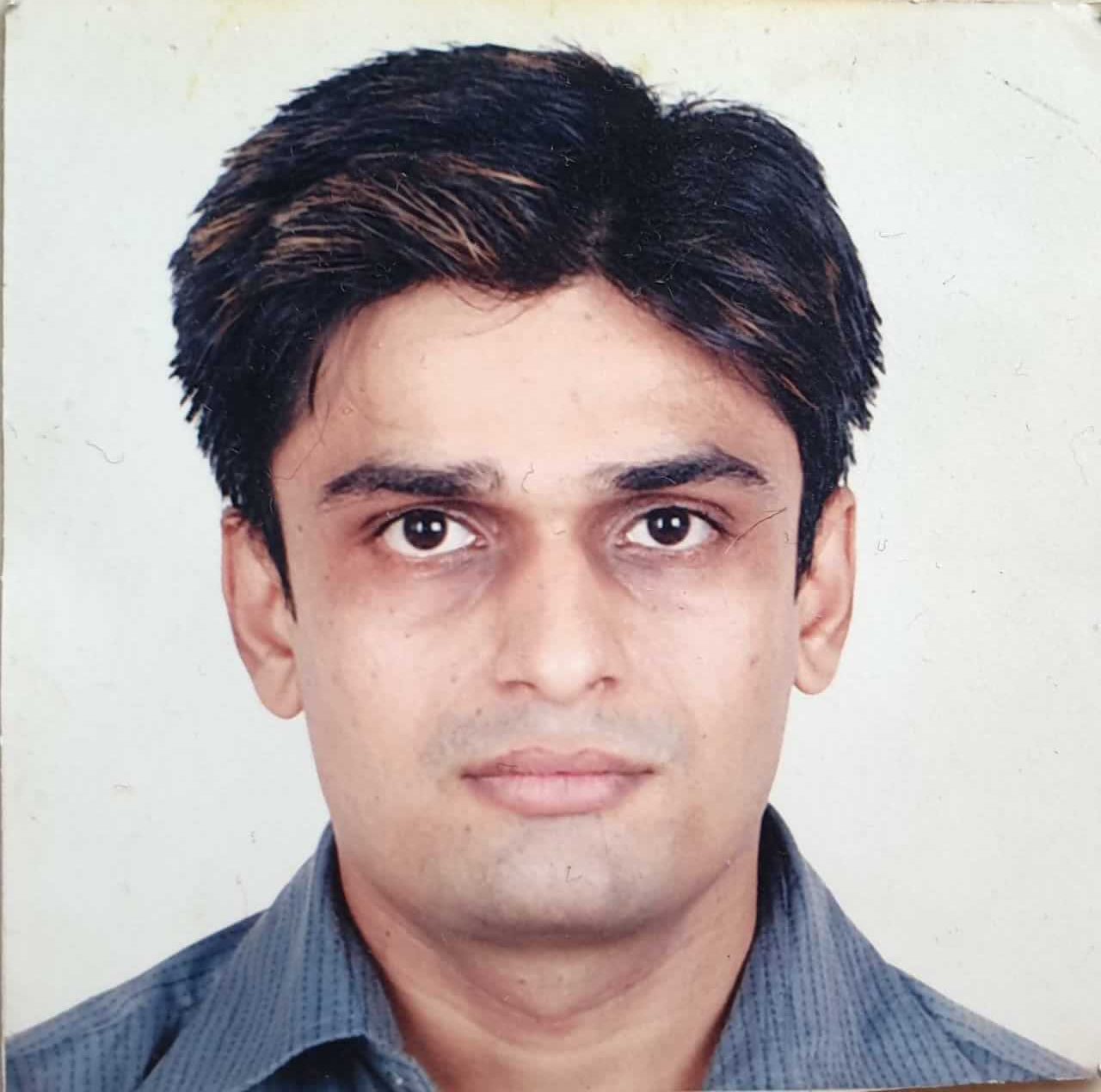
Bharat Patel
Bank of America
It is around a year since I have started my journey of learning Core Java, Advance, Hibernate and Spring.If any non-programmer want to learn Java then this place is the right option. There are many Java training institutes in city, however the thing which makes Mikado Solutions and Sanjay Sir exceptional is Quality of Teaching and connection with the students. It is sheer hardwork and excellence of Sanjay Sir to help each student to excel in career.
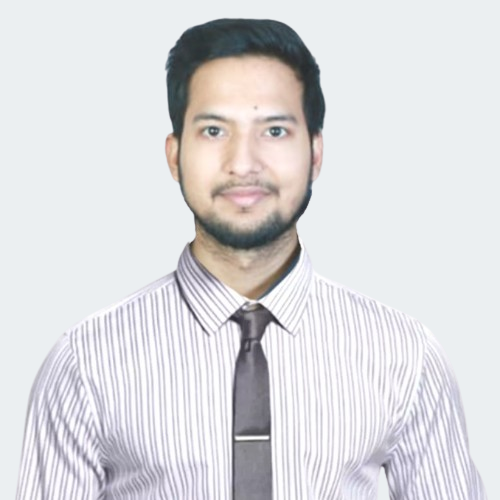
Saurabh Dubey
Morgan Stanley
It had been around 2.7 year in industry as a java developer now i can say that taking admission here for java training was the best decision taken ever … Sanjay sir’s java knowledge is vast and the way of explaining things is exceptionally great. I have completed core and advanced java in mikado and before the completion of my course i got placed.
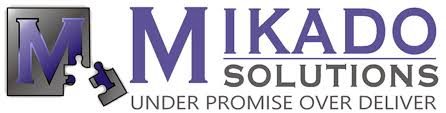